本文共 199 字,大约阅读时间需要 1 分钟。
最近在学transformer,对其中一个词embedding有疑惑,先百度翻译了一下: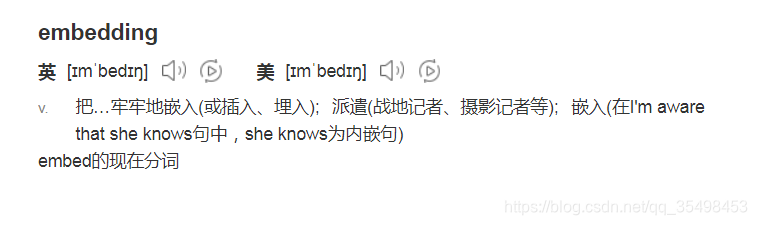
直译是嵌入的意思,然后参照了一些文献,大体总结了一下:
在深度学习里面要用向量去描述一个输入的特征,就要将输入的高维向量转化为可以代表feature的低维向量。在nlp的应用中输入一个句子,每个单词找到对应的embedding代表词向量。在视觉中输入一张图片,每个区域找到对应的embedding代表该区域。
转载地址:http://ljmsz.baihongyu.com/